Hey guys, today in this post we are going to learn about How to Create test data factory apex test class to code coverage for Quote and QuoteLineItem in Salesforce.
The @IsTest annotation Classes defined this way should only contain test methods and any methods required to support those test methods. One advantage to creating a separate class for testing is that classes defined with @IsTest don’t count against your org’s limit of 6 MB of Apex code. To know more details about Test Class, Click Here.
- Don’t forget to check out:- How to get Current Position Latitude and Longitude of Google Map in LWC. Click Here For More Information.
Final Output →
You can download file directly from github by Click Here.
Other related post that would you like to learn in Salesforce
- Find the below steps ▾
Create Apex Class Controller
Step 1:- Create Apex Class : createQuoteAndLineItemLwcCtrl.apxc
From Developer Console >> File >> New >> Apex Class
createQuoteAndLineItemLwcCtrl.apxc [Apex Class Controller]
public WITH sharing class createQuoteAndLineItemLwcCtrl {
public createQuoteAndLineItemLwcCtrl() {
}
@AuraEnabled(cacheable=TRUE)
public static List<Opportunity> getOpportunityList(Id recId){
List<Opportunity> optList = [SELECT Id, Name, Description FROM Opportunity WHERE Id=:recId];
RETURN optList;
}
//START Quote
@AuraEnabled
public static String submitOptRecord(String jsonDataStr) {
Map<String, Object> RESULT = NEW Map<String, Object>();
String DataRespoce ='';
try {
Map<String, Object> formDataMap = (Map<String, Object>)JSON.deserializeUntyped(jsonDataStr);
Map<String, Object> OptDataMap = (Map<String, Object>)formDataMap.get('optDataFyObj');
// Opportunity optObj = NEW Opportunity();
Quote optObj = NEW Quote();
optObj.Name = getStringValueFromMap(OptDataMap, 'Name');
optObj.OpportunityId = getStringValueFromMap(OptDataMap, 'OpportunityId');
optObj.Description = getStringValueFromMap(OptDataMap, 'Description');
optObj.Pricebook2Id = getStringValueFromMap(OptDataMap, 'Pricebook2Id');
List<DATABASE.SaveResult> insertResult = DATABASE.insert(NEW List<Quote>{optObj});
DataRespoce =JSON.serialize(insertResult);
}catch(Exception ex) {
RESULT.put('status', 500);
RESULT.put('message', 'Exception ' + ex.getMessage() + ',line' + ex.getLineNumber());
}
RETURN DataRespoce;
}
public static String getStringValueFromMap(Map<String, Object> dataMap, String fieldName) {
String VALUE;
try {
IF(dataMap.containsKey(fieldName)) {
VALUE = String.valueOf(dataMap.get(fieldName));
}
VALUE = String.isEmpty(VALUE) ? VALUE : String.valueOf(VALUE);
} catch(Exception ex) {
System.debug('Exception getValueFromMap : '+ ex.getMessage() + ' line ' + ex.getLineNumber());
}
RETURN VALUE;
}
public static DATE getDateValueFromMap(Map<String, Object> dataMap, String fieldName) {
DATE VALUE;
try {
String str;
IF(dataMap.containsKey(fieldName)) {
str = String.valueOf(dataMap.get(fieldName));
}
VALUE = String.isEmpty(str) ? VALUE : DATE.valueOf(str);
} catch(Exception ex) {
System.debug('Exception getIntValueFromMap : '+ ex.getMessage() + ' line ' + ex.getLineNumber());
}
RETURN VALUE;
}
//START Quote Line Item
@AuraEnabled
public static void saveQuoteLineItem(List<QuoteLineItem> quoteItemList){
try {
INSERT quoteItemList;
System.debug('quoteItemList## ' + quoteItemList);
}
catch(Exception ex) {
System.debug('Exception getValueFromMap : '+ ex.getMessage() + ' line ' + ex.getLineNumber());
}
}
//END Quote Line Item
}
Create Test Data Factory Controller
Step 2:- Create Apex Class : TestDataFactory.apxc
From Developer Console >> File >> New >> Apex Class
TestDataFactory.apxc [Apex Class Controller]
public class TestDataFactory {
public static Account createAccount() {
Account acc = NEW Account();
acc.Name='test';
acc.Description='test';
INSERT acc;
RETURN acc;
}
public static string jsonDataStrObj(){
String jsonStr =
'{"invoiceList":[' +
'{"totalPrice":5.5,"statementDate":"2011-10-04T16:58:54.858Z","lineItems":[' +
'{"Country":"India","Type":"test","Frequency":"test"},' +
'{"Country":"India","Type":"test","Frequency":"test"}],' +
'"invoiceNumber":1},' +
'{"totalPrice":11.5,"statementDate":"2011-10-04T16:58:54.858Z","lineItems":[' +
'{"Country":"India","Type":"test","Frequency":"test"},' +
'{"Country":"India","Type":"test","Frequency":"test"},' +
'{"Country":"India","Type":"test","Frequency":"test"}],"invoiceNumber":2}' +
']}';
RETURN jsonStr;
}
public static Map<String, Object> createDataMap(){
opportunity opt = NEW opportunity();
opt.Name='test';
opt.StageName='Prospecting';
opt.CloseDate=system.today();
INSERT opt;
Map<String, Object> dataMap = NEW Map<String, Object>();
dataMap.put('test1',opt);
RETURN dataMap;
}
public static opportunity createOppt(){
Account acc = TestDataFactory.createAccount();
opportunity opt = NEW opportunity();
opt.Name='test';
opt.StageName='Prospecting';
opt.CloseDate=system.today();
opt.AccountId=acc.Id;
INSERT opt;
RETURN opt;
}
public static Product2 createProduct(){
Product2 p2= NEW Product2();
p2.Name= 'test';
p2.IsActive=TRUE;
p2.ProductCode='test';
INSERT p2;
RETURN p2;
}
public static Pricebook2 createPricebook(){
Pricebook2 p1 = NEW Pricebook2();
p1.Name='test';
p1.IsActive=TRUE;
INSERT p1;
RETURN p1;
}
public static PricebookEntry createPricebookEntry(){
Id priceId = Test.getStandardPricebookId();
Pricebook2 p1 = TestDataFactory.createPricebook();
Product2 p2 = TestDataFactory.createProduct();
PricebookEntry priceBkEntry = NEW PricebookEntry();
priceBkEntry.UseStandardPrice=TRUE;
priceBkEntry.Pricebook2Id= priceId;
//priceBkEntry.Pricebook2Id=p1.Id;
priceBkEntry.Product2Id=p2.id;
priceBkEntry.UnitPrice=40446;
priceBkEntry.UseStandardPrice=FALSE;
priceBkEntry.IsActive=TRUE;
//NEW PricebookEntry (Product2Id=p2.id,Pricebook2Id=Test.getStandardPricebookId(),UnitPrice=40446, UseStandardPrice=TRUE, isActive=TRUE);
INSERT priceBkEntry;
RETURN priceBkEntry;
}
public static Quote createQuote(){
Id priceId = Test.getStandardPricebookId();
Opportunity opt = TestDataFactory.createOppt();
Pricebook2 p1= TestDataFactory.createPricebook();
Quote q2 = NEW Quote();
q2.Email='test@test.com';
q2.Name='test';
q2.OpportunityId=opt.Id;
//q2.Pricebook2Id=p1.Id;
q2.Pricebook2Id=priceId;
INSERT q2;
RETURN q2;
}
public static List<QuoteLineItem> createQuoteLineItem(){
Id priceId = Test.getStandardPricebookId();
List<QuoteLineItem> quoteItemLst = NEW List<QuoteLineItem>();
Quote q2= TestDataFactory.createQuote();
PricebookEntry priceBkEntry= TestDataFactory.createPricebookEntry();
Product2 p2= TestDataFactory.createProduct();
QuoteLineItem qitm = NEW QuoteLineItem();
qitm.Description='test';
qitm.QuoteId=q2.Id;
qitm.PricebookEntryId=priceBkEntry.Id;
qitm.Quantity=1.0;
qitm.UnitPrice=1.0;
qitm.Product2Id=p2.Id;
INSERT qitm;
quoteItemLst.add(qitm);
RETURN quoteItemLst;
}
}
Create Apex Test Class
Step 3:- Create Apex Class : createQuoteAndLineItemLwcCtrlFactoryTest.apxc
From Developer Console >> File >> New >> Apex Class
createQuoteAndLineItemLwcCtrlFactoryTest.apxc [Apex Class Controller]
@isTest
public class createQuoteAndLineItemLwcCtrlFactoryTest {
static testMethod void getOpportunityListTest(){
opportunity optObj = TestDataFactory.createOppt();
test.startTest();
createQuoteAndLineItemLwcCtrl.getOpportunityList(optObj.Id);
test.stopTest();
}
static testMethod void submitOptRecordTest(){
String jsonDataStr = TestDataFactory.jsonDataStrObj();
test.startTest();
createQuoteAndLineItemLwcCtrl.submitOptRecord(jsonDataStr);
test.stopTest();
}
static testMethod void getDateValueFromMapTest(){
Map<String, Object> dataMap = TestDataFactory.createDataMap();
test.startTest();
createQuoteAndLineItemLwcCtrl.getDateValueFromMap(dataMap,'test');
test.stopTest();
}
static testMethod void saveQuoteLineItemTest(){
//Id priceId = Test.getStandardPricebookId();
List<QuoteLineItem> quotItmLst = TestDataFactory.createQuoteLineItem();
test.startTest();
createQuoteAndLineItemLwcCtrl.saveQuoteLineItem(quotItmLst);
test.stopTest();
}
}
Further post that would you like to learn in Salesforce
What is Apex test class in Salesforce?
Test classes are written in Apex and are used to verify that the code written by developers works as intended before it is deployed to a production environment. Test classes are not counted in code coverage before they get deployed by the Salesforce Admin in final production.
What is Apex Test Suite in Salesforce?
A test suite is a collection of Apex test classes that you run together. For example, create a suite of tests that you run every time you prepare for a deployment or Salesforce releases a new version. Set up a test suite in the Developer Console to define a set of test classes that you execute together regularly.
What is test data for apex tests?
est data is the transient data that is not committed to the database and is created by each test class to test the Apex code functionality for which it is created. The use of this transient test data makes it easy to test the functionality of other Apex classes and triggers.
Related Topics | You May Also Like
Note:: – You will get an email, so put correct email and mobile number and BEGIN YOUR JOURNEY from Today!
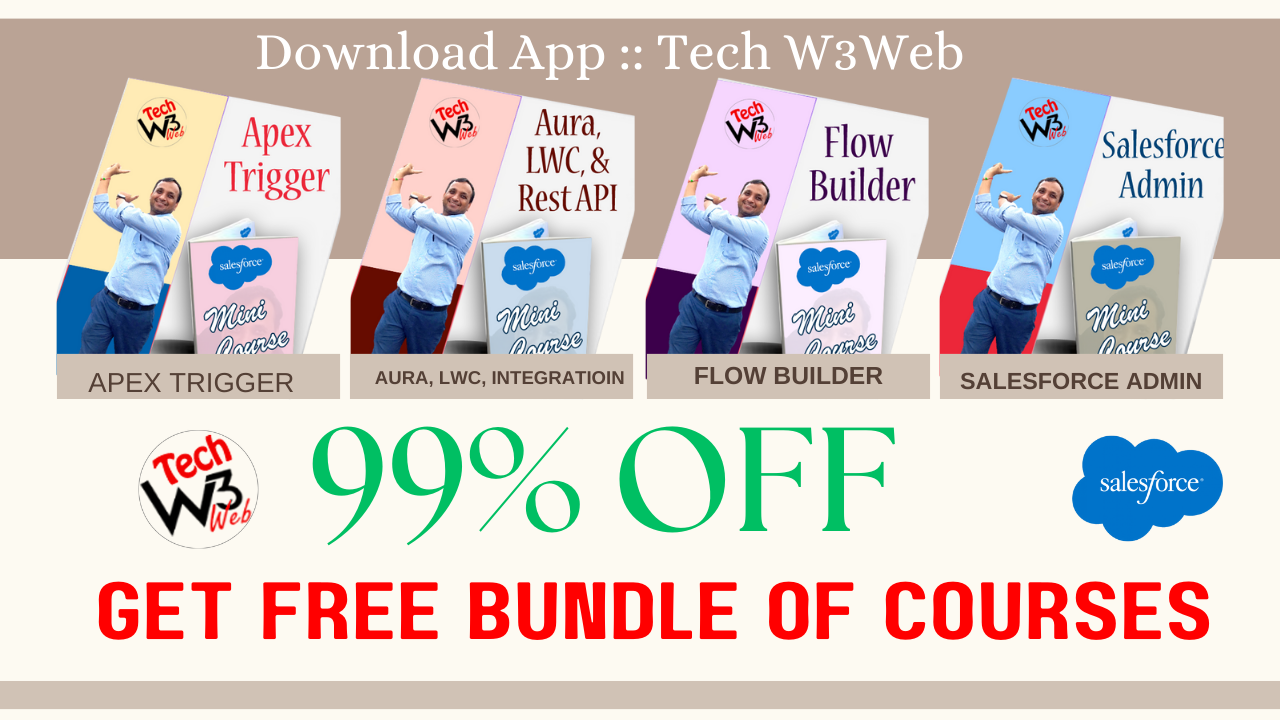
Our Free Courses →
👉 Get Free Course →
![]() 📌 Salesforce Administrators 📌 Salesforce Lightning Flow Builder 📌 Salesforce Record Trigger Flow Builder |
👉 Get Free Course →
![]() 📌 Aura Lightning Framework 📌 Lightning Web Component (LWC) 📌 Rest APIs Integration |